Check Image Height Nd Width Before Uploading a Image
Image resizing is computationally expensive and usually done on the server-side so that right-sized epitome files are delivered to the client-side. This arroyo also saves data while transmitting images from the server to the customer.
However, there are a couple of situations where you might need to resize images purely using JavaScript on the client side. For case -
- Resizing images before uploading to server
Uploading a large file on your server will take a lot of time. You tin can first resize images on the browser so upload them to reduce upload fourth dimension and meliorate application performance.
- Rich image editors that piece of work on client-side
A rich image editor that offers prototype resize, ingather, rotation, zoom IN and zoom OUT capabilities often require prototype manipulation on the client-side. The speed is critical for the user in these editors.
If a user is manipulating a heavy paradigm, it will take a lot of time to download transformed images from the server. Imagine this with operations similar undo/redo and complex text and prototype overlays.
Image manipulation in JavaScript is washed using the canvas element. There are libraries like cloth.js that offer rich APIs.
Apart from the above two reasons, in almost all cases, you would want to get the resized images from the backend itself and then that customer doesn't have to bargain with heavy processing tasks.
In this post-
- We will first talk nearly how to do resizing purely in JavaScript using the
canvas
element.
- Then we will encompass in great item how you can resize, crop, and do a lot with images by changing the image URL in the
src
attribute. This is the preferred manner to resize images without degrading the user experience programmatically.Also, nosotros will acquire how you tin can do this without needing to set any libraries or backend servers.
Image resizing in JavaScript - Using sheet element
The HTML <canvas>
element is used to draw graphics, on the fly, via JavaScript. Resizing images in browser using canvas
is relatively simple.
drawImage
function allows us to render and scale images on canvas element.
drawImage(prototype, ten, y, width, top)
The get-go argument epitome
can be created using the Image()
constructor, equally well as using whatever existing <img>
element.
Let's write the code to resize a user-uploaded image on the browser side 300x300
.
<html> <body> <div> <input blazon="file" id="epitome-input" have="image/*"> <img id="preview"></img> </div> <script> permit imgInput = document.getElementById('image-input'); imgInput.addEventListener('change', function (e) { if (e.target.files) { let imageFile = e.target.files[0]; var reader = new FileReader(); reader.onload = function (e) { var img = certificate.createElement("img"); img.onload = office (event) { // Dynamically create a sail element var sheet = certificate.createElement("canvas"); // var sheet = document.getElementById("canvas"); var ctx = canvas.getContext("second"); // Bodily resizing ctx.drawImage(img, 0, 0, 300, 300); // Bear witness resized image in preview chemical element var dataurl = canvass.toDataURL(imageFile.type); certificate.getElementById("preview").src = dataurl; } img.src = e.target.issue; } reader.readAsDataURL(imageFile); } }); </script> </body> </html>
Let's empathise this in parts. First, the input file type field in HTML
<html> <torso> <div> <input type="file" id="image-input" accept = "paradigm/*"> <img id="preview"></img> </div> </trunk> </html>
Now we demand to read the uploaded epitome and create an img
element using Paradigm()
constructor.
let imgInput = document.getElementById('image-input'); imgInput.addEventListener('modify', function (eastward) { if (e.target.files) { let imageFile = e.target.files[0]; var reader = new FileReader(); reader.onload = function (east) { var img = document.createElement("img"); img.onload = role(consequence) { // Actual resizing } img.src = e.target.event; } reader.readAsDataURL(imageFile); } });
Finally, let's draw the epitome on canvas and show preview chemical element.
// Dynamically create a canvas element var sail = document.createElement("sail"); var ctx = canvas.getContext("2nd"); // Actual resizing ctx.drawImage(img, 0, 0, 300, 300); // Show resized image in preview element var dataurl = canvas.toDataURL(imageFile.type); certificate.getElementById("preview").src = dataurl;
You might observe that the resized image looks distorted in a few cases. It is because we are forced 300x300
dimensions. Instead, nosotros should ideally just manipulate i dimension, i.e., meridian or width, and adjust the other accordingly.
All this can be done in JavaScript, since you have admission to input image original peak (img.width
) and width using (img.width
).
For case, we tin fit the output image in a container of 300x300
dimension.
var MAX_WIDTH = 300; var MAX_HEIGHT = 300; var width = img.width; var height = img.height; // Change the resizing logic if (width > height) { if (width > MAX_WIDTH) { height = height * (MAX_WIDTH / width); width = MAX_WIDTH; } } else { if (top > MAX_HEIGHT) { width = width * (MAX_HEIGHT / top); height = MAX_HEIGHT; } } var canvass = certificate.createElement("canvas"); sail.width = width; canvas.meridian = acme; var ctx = canvas.getContext("2nd"); ctx.drawImage(img, 0, 0, width, top);
Decision-making image scaling behavior
Scaling images can consequence in fuzzy or blocky artifacts. There is a trade-off between speed and quality. By default browsers are tuned for better speed and provides minimum configuration options.
You tin play with the post-obit properties to control smoothing outcome:
ctx.mozImageSmoothingEnabled = simulated; ctx.webkitImageSmoothingEnabled = false; ctx.msImageSmoothingEnabled = false; ctx.imageSmoothingEnabled = false;
Image resizing in JavaScript - The serverless way
ImageKit allows you lot to manipulate image dimensions directly from the image URL and become the verbal size or crop y'all want in real-fourth dimension. Showtime with a single principal epitome, equally large as possible, and create multiple variants from the same.
For case, nosotros can create a 400 x 300
variant from the original image like this:
https://ik.imagekit.io/ikmedia/ik_ecom/shoe.jpeg?tr=w-400,h-300
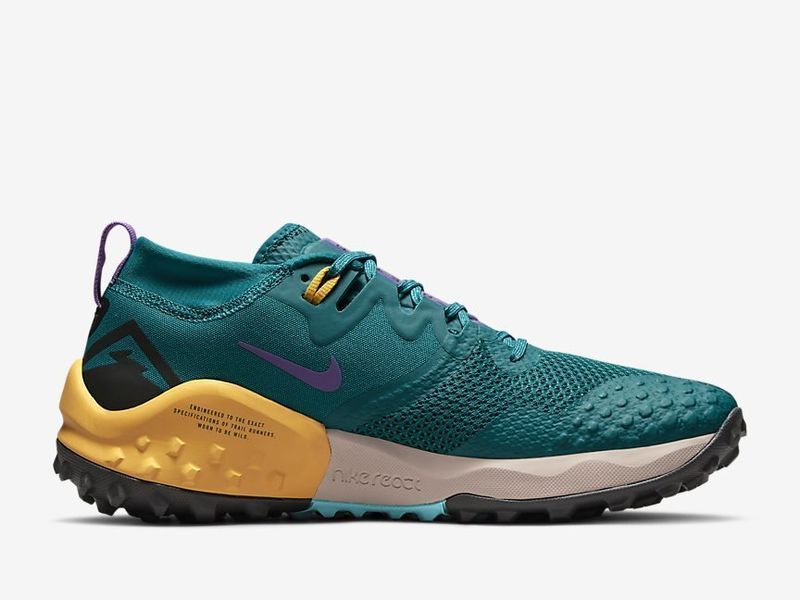
You tin utilise this URL directly on your website or app for the product image, and your users become the correct prototype instantly.
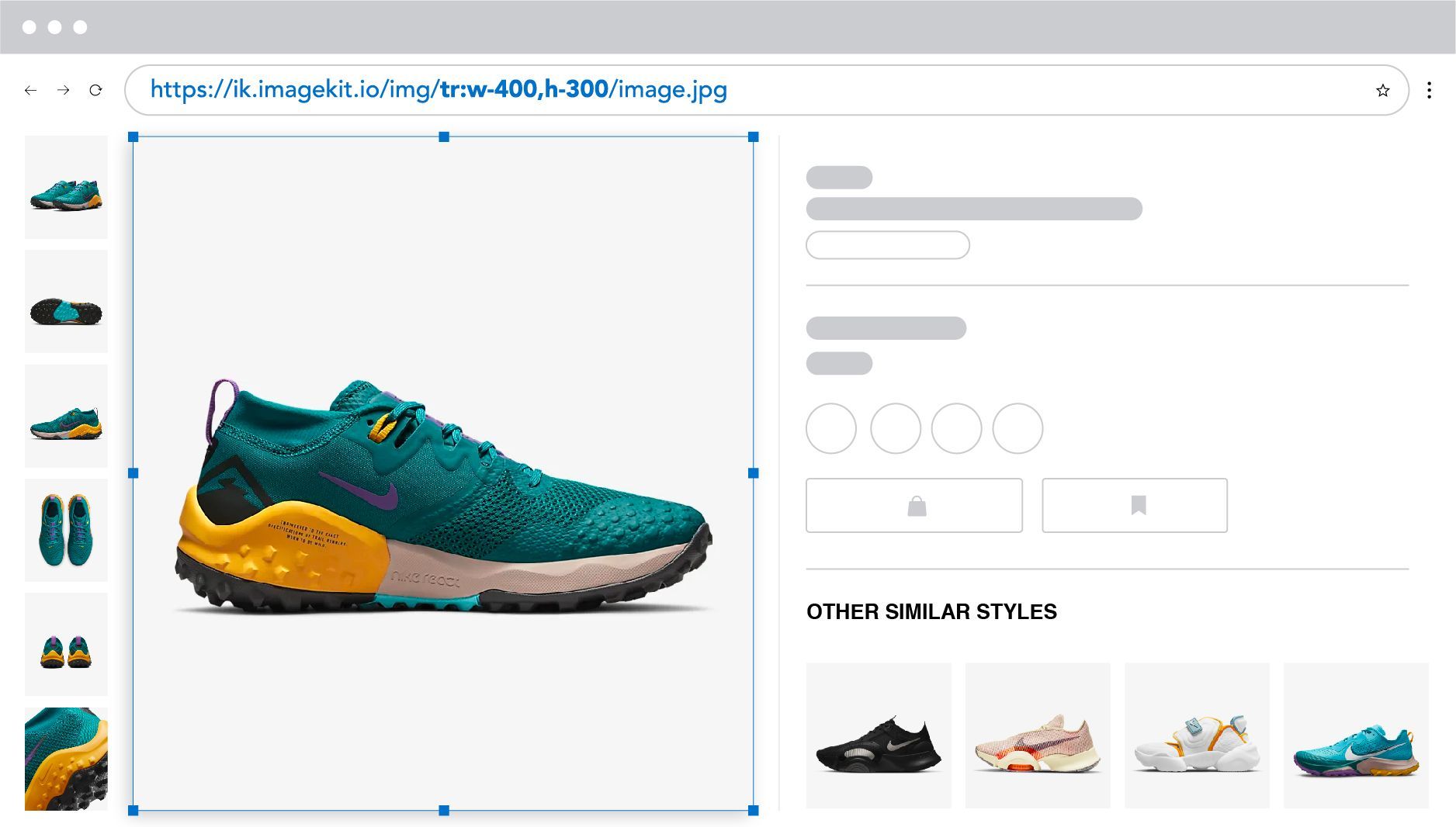
If yous don't want to crop the image while resizing, at that place are several possible crop modes.
https://ik.imagekit.io/ikmedia/ik_ecom/shoe.jpeg?tr=due west-400,h-300,cm-pad_resize,bg-F5F5F5
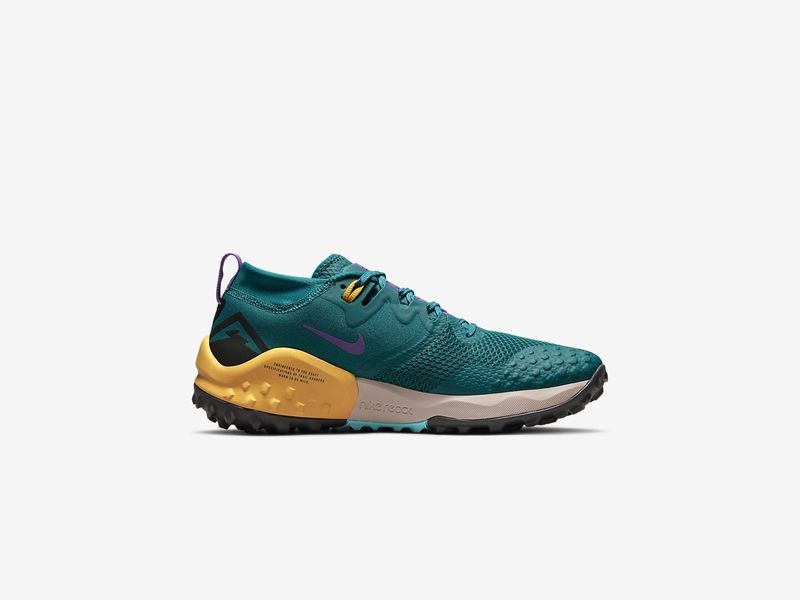
We accept published guides on how you can do the following things using ImageKit'due south real-fourth dimension image manipulation.
- Resize epitome - Basic summit & width manipulation
- Cropping & preserving the aspect ratio
- Face and object detection
- Add together a watermark
- Add a text overlay
- Adapt for slow internet connection
- Loading a blurred low-quality placeholder
Summary
- In most cases, you should not do image resizing in the browser because it is boring and results in poor quality. Instead, you should utilize an epitome CDN like ImageKit.io to resize images dynamically by changing the image URL. Try our forever gratuitous program today!
- If your use-example demands client-side resizing, it is possible using the
canvas
element.
Source: https://imagekit.io/blog/how-to-resize-image-in-javascript/
0 Response to "Check Image Height Nd Width Before Uploading a Image"
Post a Comment